Entity Framework Models
In Entity Framework Core (EF Core), a model is a collection of entity classes that represent the domain of your application and a context object. It defines the data structure and describes how it maps to database objects, such as tables and views, and their relationships. Entity classess in EF Core are typically represented by POCO (Plain Old CLR Objects), with configurations applied either through data annotations or the Fluent API.
1public class Inventory
2{
3 public int Id { get; set; }
4 public string Name { get; set; }
5 public string SKU { get; set; }
6 public int Quantity { get; set; }
7}
Register POCO type to the model.
In EF Core you can register POCO objects as part of the model to represent the entity in the database. An entity is the C# representation of a database table (or view, or other database objects).
There are two common approaches to register POCO to the model:
1. Using DbSet<T> in the DbContext
One way to create an entity from a POCO object is to add the POCO type as a DbSet<T> property in the DbContext. This approach automatically registers the type as part of the model.
1public class StoreContext: DbContext
2{
3 public DbSet<Inventory> Inventories { get; set; }
4}
5
2. Using the OnModelCreating Method
Another approach is to explicitly specify the entity in the OnModelCreating method. This is useful when you need additional control or when you do not want to expose the entity as a DbSet<T> property.
1public class StoreContext: DbContext
2{
3 public DbSet<Inventory> Inventory { get; set; }
4
5 protected override void OnModelCreating(ModelBuilder modelBuilder)
6 {
7 modelBuilder.Entity<Employee>();
8 }
9}
10
How these POCO classes translate to database objects (tables, views or stored procedures) is dictated by a set of conventions. You can override any convention with the help of data annotations (attributes you place over the classes and properties) or using the Fluent API.
1public class MyContext: DbContext
2{
3 public DbSet<Inventory> Inventories { get; set; }
4}
5
6[Table("StoreInventory")]
7public class Inventory
8{
9 public int Id { get; set; }
10 public string Name { get; set; }
11 [Required]
12 public string SKU { get; set; }
13 public int Quantity { get; set; }
14}
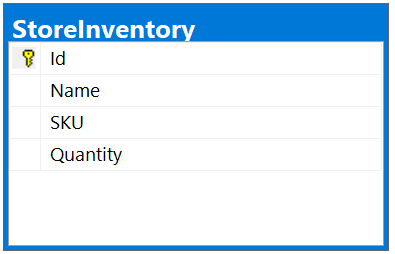
In the example above, the table naming convention dictates that the table name will match the name of the DbSet (Inventories). However, by adding the [Table("StoreInventory")] attribute to the model class, we override this convention and explicitly set the table name to StoreInventory.
The required attribute will result in a column that doesn’t accept null values. The column will accept empty strings. If you don’t want empty strings to be accepted too you can use [MinLength(1)]
Homework
Section | Assignment name |
---|---|
Models | Assignment N1 - Create your first EF core project |