Create Entity Framework Core with SQL Server project
An EF Core project is a C# class library project with nuget packages for Microsoft.EntityFrameworkCore.SqlServer and Microsoft.EntityFrameworkCore.Tools installed. After the installation you will be able to create an application DbContext and Entities.
Create your first EF core project.
You are creating an application for a small store chain. The chain has several store locations. The application you are building will handle the daily store procedures and inventory. As a developer, your task is to create a new EF Core project. The project will include a Store table. The Store table must have Id, Location, and Name columns. Create the table with the described columns.
Before you start you will need to have the following software and frameworks installed:
- IDE: Visual Studio 2022 or later Free Developer Offers
- .NET Framework: .NET 8 Download the SDK
- Database and SSMS: SQL Server Express Download Server, Downlaod SSMS
- ORM: EF Core 9
Project structure
- SupermarketDomain (class library)
- SupermarketDesignProject (console application)
SupermarketDomain - configure entity framework in .net core
For simplicity of the example I put both the DBContext and the models in the same project. You could create separate projects for both of them if you feel the separation is necessary.
1. Create a new Class library project called SupermarketDomain.
2. Using NuGet package manager install EntityFrameworkCore.SQLServer
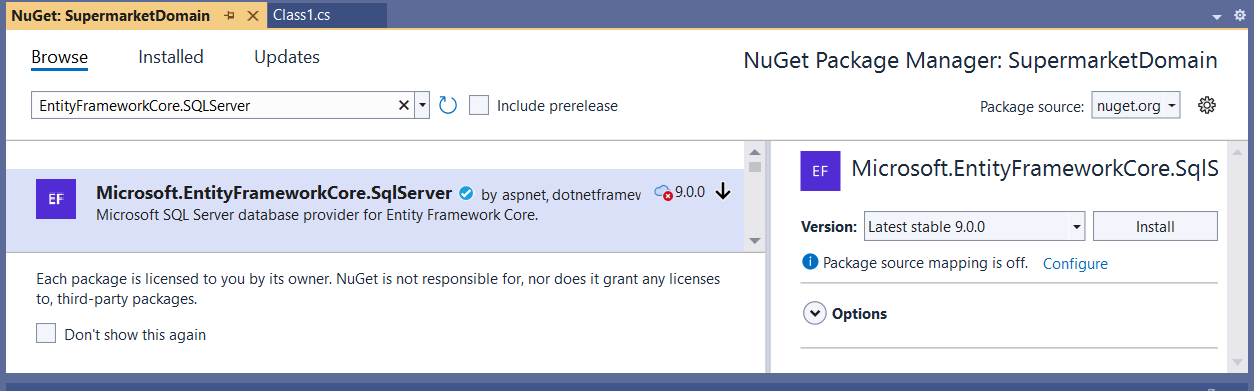
3. Create one new class SupermarketContext.cs to hold the database context. The class must inherit from DbContext.
3.1. Create one constructor that accepts DbContextOptions<SupermarketContext> options and passes it to its base. This is important so that the registration of the db context to the dependency injection works.
3.2. Create one more empty constructor.
3.3. Override OnConfiguring method and set a default connection string
1public class SupermarketContext : DbContext
2{
3 public SupermarketContext(DbContextOptions<SupermarketContext> options) : base(options)
4 {
5 }
6
7 public SupermarketContext()
8 {
9 }
10
11 protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder)
12 {
13 optionsBuilder.UseSqlServer("Server=localhost\SQLEXPRESS;
14 Initial Catalog=SuperMarket_Dev;Integrated Security=True;TrustServerCertificate=True;");
15 base.OnConfiguring(optionsBuilder);
16 }
17}
4. Defining the Model - Create a second class called Store. This will represent the chain stores and will be represented as a table inside the database. By convention the Id property will be the table key and is auto incremented.
1public class Store
2 {
3 public int Id { get; set; }
4 public string Name { get; set; }
5 public string Location { get; set; }
6 }
5. Last step is registering the new Entity model inside the DBContext. We do that by creating one new DBSet collection property as follows:
1public class SupermarketContext : DbContext
2{
3 public DbSet<Store> Stores { get; set; }
4
5 public SupermarketContext(DbContextOptions<SupermarketContext> options) : base(options)
6 {
7 }
8
9 public SupermarketContext()
10 {
11 }
12
13 protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder)
14 {
15 optionsBuilder.UseSqlServer("Server=localhost\SQLEXPRESS;
16 Initial Catalog=SuperMarket_Dev;Integrated Security=True;TrustServerCertificate=True;");
17 base.OnConfiguring(optionsBuilder);
18 }
19}
Here the name of the property will be the name of the table.
Setup the SupermarketDesignProject
1. Create console applications that will be used as a host for the EF design tools
2. Using NuGet install EntityFrameworkCore.Design

3. Set the project as a Startup project
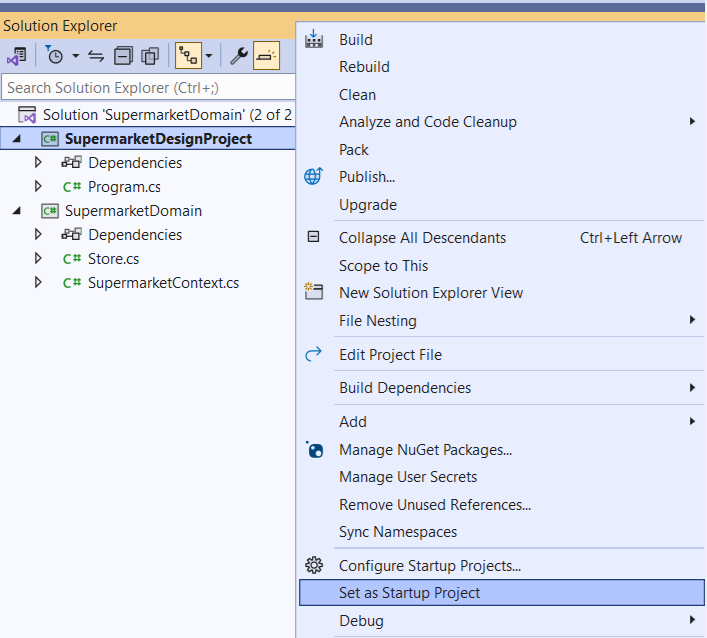
4. Go to the package manager Console and select the default project to be SupermarketDomain
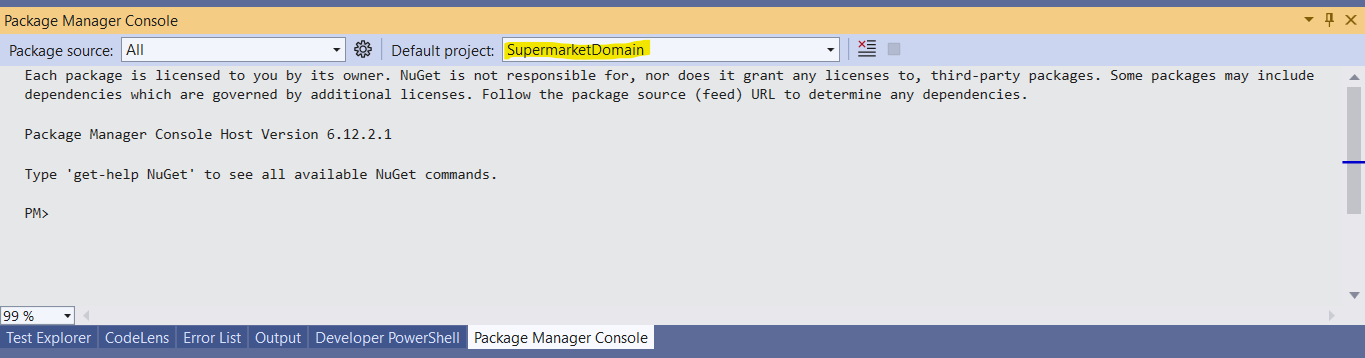
5. In SupermarketDesignProject add a reference to SupermarketDomain project
6. In the SupermarketDomain project, create a DesignTimeDbContextFactory class. This class is specifically designed for use at design time. It will provide the DbContext configuration, including the connection string, to specify the server and database where migration scripts should be executed.
1public class DesignTimeDbContextFactory : IDesignTimeDbContextFactory<SupermarketContext>
2{
3 public SupermarketContext CreateDbContext(string[] args)
4 {
5 var optionsBuilder = new DbContextOptionsBuilder<SupermarketContext>();
6 optionsBuilder.UseSqlServer("Server=localhost\SQLEXPRESS;
7 Initial Catalog=SuperMarket_Dev;Integrated Security=True;TrustServerCertificate=True;");
8
9 return new SupermarketContext(optionsBuilder.Options);
10 }
11 }
7. Rebuild the solution and make sure there are no errors
8. From PMC (package manager console) run Install-Package Microsoft.EntityFrameworkCore.Tools
9. From PMC run Add-Migration Initial. This will create a Migration folder with the new migration script file.
10. To apply this migration script to your database run Update-Database. This willcreate a sql script under the hood and run it on your server. If there isn't a databse create it will create it.
11. Open SSMS and search for your newly created database and table.
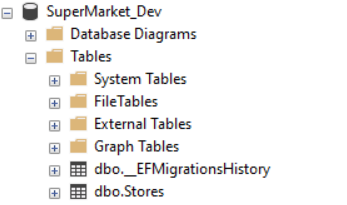
Every time you make changes to the Domain (the tables) you will have to create one new migration script by running Add-Migration MigrationName. And as a second step you must run the Update-Database so that the migration script is executed over the database.