Generated values
Generated values are values that will be computed or generated by the database. Here are a few ways you can create such columns.
Auto incremented primary key
By convention, any primary key column of type short, int, long, or Guid is configured as an auto incrementing identity column. When a new record is inserted, a unique value is automatically generated for it.
1public class School
2{
3 public int SchoolId { get; set; } // this is auto incremented achiving unique values for the primary key
4}
5
1public class Student
2{
3 public Guid Id { get; set; } // this is auto generated with unique values
4}
5
If we want to use any other name for primary key name we must decorate the property with the [Key] attribute from System.ComponentModel.DataAnnotations. This will tell EF Core that this specific property is the primary key
1 public class Supplier
2 {
3 [Key]
4 public int SupplierKey { get; set; }
5 public string Name { get; set; }
6 }
7
As you can see in the migration script, the SupplierKey property needs an identity for the table.
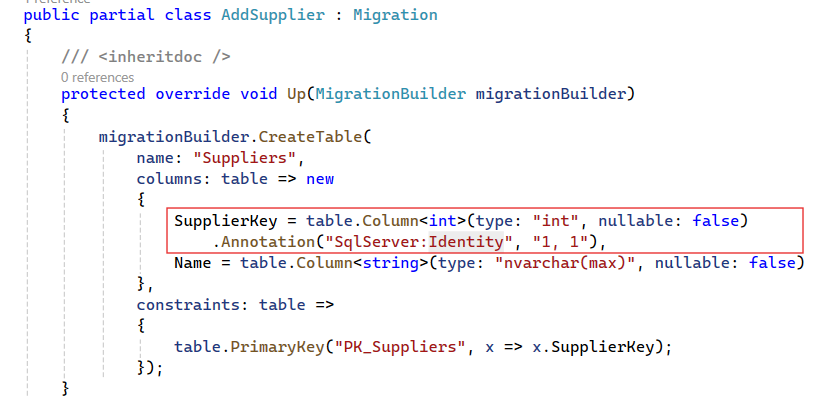
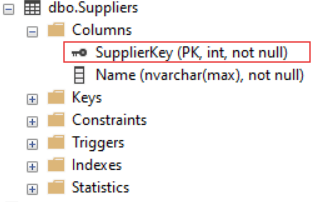
Computed columns
Computed values are values that the database will calculate based on other columns in the same table. Databases don’t allow specifying functions like GETDATE() in computed columns.
1public class Store
2{
3 public int Id { get; set; }
4 public string Name { get; set; }
5 public string Location { get; set; }
6 public List<Inventory> Inventory{ get; set; }
7 public string FullInfo { get; set; }
8}
9
10protected override void OnModelCreating(ModelBuilder modelBuilder)
11{
12 modelBuilder.Entity<Store>(entity => {
13 entity.Property(e => e.FullInfo)
14 .HasComputedColumnSql("[Name] + ', ' + [Location]");
15 });
16}
17
This is a virtual computed column. It will be calculated every time it is fetched from the database. You can see the computed column in the database as well.
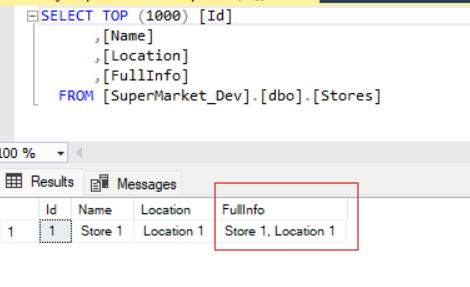
If you want the column's value to be stored in the database, you can set the stored parameter to true in the HasComputedColumnSql method. This ensures the column is materialized and automatically recalculated whenever the data it depends on is updated.
1public class Store
2{
3 public int Id { get; set; }
4 public string Name { get; set; }
5 public string Location { get; set; }
6 public List<Inventory> Inventory{ get; set; }
7 public string FullInfo { get; set; }
8}
9
10 protected override void OnModelCreating(ModelBuilder modelBuilder)
11 {
12 modelBuilder.Entity<Store>(entity => {
13 entity.Property(e => e.FullInfo)
14 .HasComputedColumnSql("[Name] + ', ' + [Location]", true);
15 });
16 }
17
Default values
A default value is automatically assigned to a column when no explicit value is provided in the insert statement.
1protected override void OnModelCreating(ModelBuilder modelBuilder)
2{
3 modelBuilder.Entity<Store>().Property(c => c.OpenHours).HasDefaultValue(24); // Sets default value to 24h
4}
5
Date/time value generation
You can call SQL fragments as a default value parameter.
1protected override void OnModelCreating(ModelBuilder modelBuilder)
2{
3 modelBuilder.Entity<Inventory>().Property(i=>i.CreatedDate).HasDefaultValueSql("GETDATE()");
4}
Unique constraint
To create a unique constraint for your database you create an index and set the isQnique value to true. This will force each record in the database to be unique.
1 [Index(nameof(Name), IsUnique = true)]
2 public class Supplier
3 {
4 [Key]
5 public int SupplierKey { get; set; }
6 public string Name { get; set; }
7 }