Entity framework core add entity
Inserting a new entity is done following these 3 steps:
- Create new Entity object
- Add the Entity object to the DBContext
- Call DBContext.SaveChanges()
Let's look at them in more details:
Create new Entity object
For our example I will add one new inventory item to the database. From the Entity class you can see that the Store id is a required field
public class Inventory
{
[Key]
public int InventoryId { get; set; }
public string Name { get; set; }
public Store Store { get; set; }
public int StoreId { get; set; }
public DateTime CreatedDate { get; set; }
}
It happens for me to know that I have a store with an id = 1 in my database. This is the store that I will be using.
var inventory = new Inventory() { Name = "Candy", StoreId=1 };
Add the Entity object to the DBContext
There are several ways to add an entry to your DBContext
2.1. Adding a single item using context.Entity.Add(entity) or context.Add(entity)
Both methods will add the entity to the db context with a state “Added”
var context = new SupermarketContext();
using (context)
{
var inventory = new Inventory() { Name = "Candy", StoreId = 1 };
context.Inventories.Add(inventory);
context.SaveChanges();
var inventory2 = new Inventory() { Name = "Candy mint", StoreId = 1 };
context.Add(inventory);
context.SaveChanges();
}
The Id of the inventory item is not specified, because it is an auto increment field and will be filled by the database.
2.2. Add a range of items at once using context.Entity.AddRange(entity[]) or context.AddRange(entity[])
Both methods will add a collection of entity properties
var context = new SupermarketContext();
using (context)
{
var inventory = new Inventory() { Name = "Candy", StoreId = 1 };
var inventory2 = new Inventory() { Name = "Candy mint", StoreId = 1 };
context.AddRange(new[] { inventory, inventory2 });
context.SaveChanges();
}
2.3. Add the full graph of a new entity
You can add linked objects at once. In the example two inventory items are added at the same time as the store entity. Only the Store entity is marked as added to the context (context.Stores.Add(supermarket);) but the context will traverse the full entity graph and figure out that both inventory items are also new.
var context = new SupermarketContext();
using (context)
{
var supermarket = new Store()
{
Name = "Store 3",
Location = "Location 3",
Inventory = new List<Inventory>() {
new Inventory(){Name="Bread"},
new Inventory(){Name="Tomatoes"}
}
};
context.Stores.Add(supermarket);
context.SaveChanges();
}

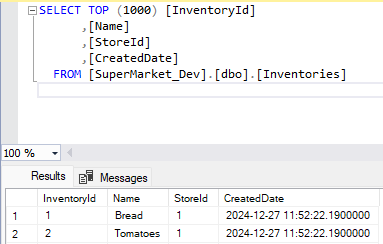
Even when the store id foreign key is not specified inside any of the inventory objects, entity framework would link the records together and the storeId column would be populated with the newly created store id.
2.4. Add an item using context.Entity.Attach(entity[]) or context.Attach(entity[])
When you attach an entity without an id it will be automatically added to the change tracker with the state Added
var context = new SupermarketContext();
using (context)
{
var inventory = new Inventory() { Name = "Bonbons", StoreId = 1 };
context.Inventories.Attach(inventory);
context.SaveChanges();
}
Call DBContext.SaveChanges()
After you have added the entity to the context you need to call SaveChanges() in order for the changes to be saved into the database.