Entity Framework Core getting started
What is Entity Framework core?
Entity Framework Core (EF Core) is a modern Object-Relational Mapping (ORM) framework designed to simplify database interactions in .NET applications. With EF Core, developers can work with data using familiar C# classes and objects, eliminating the need to write complex SQL queries. Whether you're building a new application or integrating with an existing database, EF Core offers powerful tools to streamline your workflow.
Object-Relational Mapping (ORM)
ORM is a programming technique that:
- Maps database tables to classes in a programming language.
- Converts rows into objects and columns into properties.
- Manages relationships between objects based on database relationships.
EF Core Versions
EF Core Version | Release Date | Target Framework |
---|---|---|
EF Core 9.0 | Nov 2024 | .NET 8 |
EF Core 8.0 | Nov 2023 | .NET 8 |
EF Core 7.0 | Nov 2022 | .NET 6 |
EF Core 6.0 | Nov 2021 | .NET 6 |
EF Core 5.0 | Nov 2020 | .NET Standard 2.1 |
EF Core 3.0 | Sept 2019 | .NET Standard 2.1 |
EF Core 2.0 | August 2017 | .NET Standard 2.0 |
EF Core 1.0 | June 2016 | .NET Framework 4.5.1, .NET Standard 1.3 |
Features of EF Core
- Simplified Database Access: Eliminates the need for complex SQL queries or manual connection code.
- Cross-Platform Compatibility: Works seamlessly on Windows, Linux, and macOS.
- Broad Database Support:
- Relational: Azure SQL, MSSQL, MySQL, PostgreSQL, MariaDB, Oracle DB, SQLite, DB2.
- Non-relational: Cosmos DB, MongoDB.
- CRUD Operations: Built-in methods for Create, Read, Update, and Delete operations on table data.
- Code-First Development: Tools to generate database structures from C# code.
- LINQ Queries: Querying a database could be done with the help of LINQ expressions. The expressions are translated to database-specific scripts. EF Core also has a built-in mechanism to execute SQL database-specific scripts.
- Concurrency: EF Core implements optimistic concurrency. Optimistic concurrency assumes conflicts rarely occur. There are no locks in optimistic concurrency, but EF arranges for the modification to fail on save if data has been modified since it was pulled.
- Compiled Queries: EF Core allows you to create compiled queries for better performance. Compiled queries are cached and reused, avoiding the overhead of query parsing and translation on subsequent executions.
- Interceptors: EF Core provides interceptors to hook into database operations like queries, commands, or saving changes.
- Temporal Tables: EF Core supports temporal tables for historical data tracking. Allows querying historical versions of data, ideal for audit trails or debugging.
- Raw SQL Interpolation: EF Core allows parameterized raw SQL queries, improving readability and security.
- Advanced Features: Change tracking, schema migrations, and more.
Development Approaches
EF core code first Approach
This approach is ideal for new applications. Developers write C# code to define the database structure, and EF Core automatically generates the corresponding database tables. Changes to the database schema—such as creating, altering, or dropping tables, adding indexes, and defining foreign keys—are managed with the CLI Migration tool. Using the 'migration add' command, EF Core detects changes in the DbContext and Entity classes and creates a migration file with the necessary sequential updates for the database.
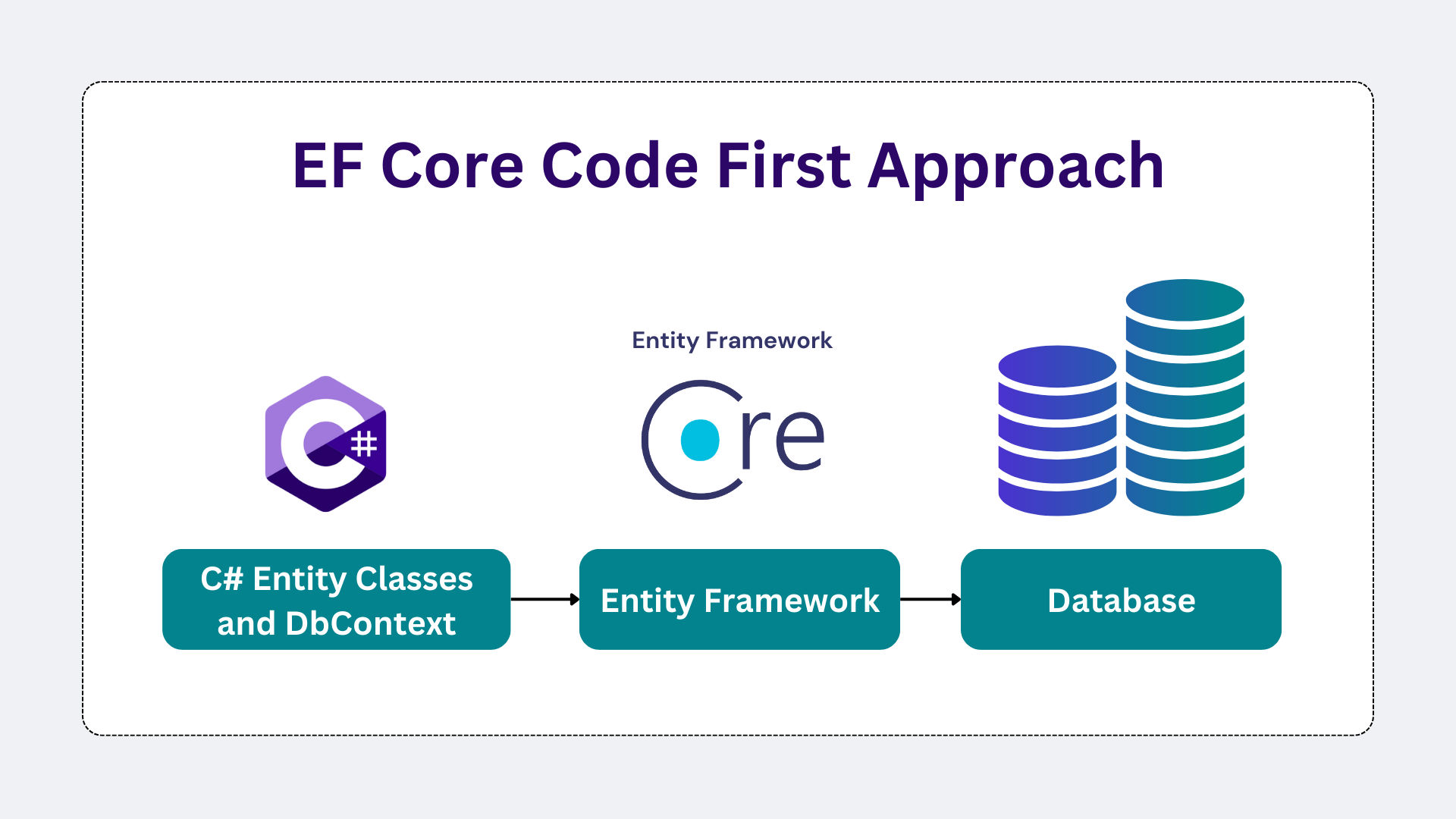
EF core database first Approach
For existing databases, EF Core can generate C# models and a DbContext class that map to the database structure. The dbcontext scaffold tool automates this process, making it quick and efficient.
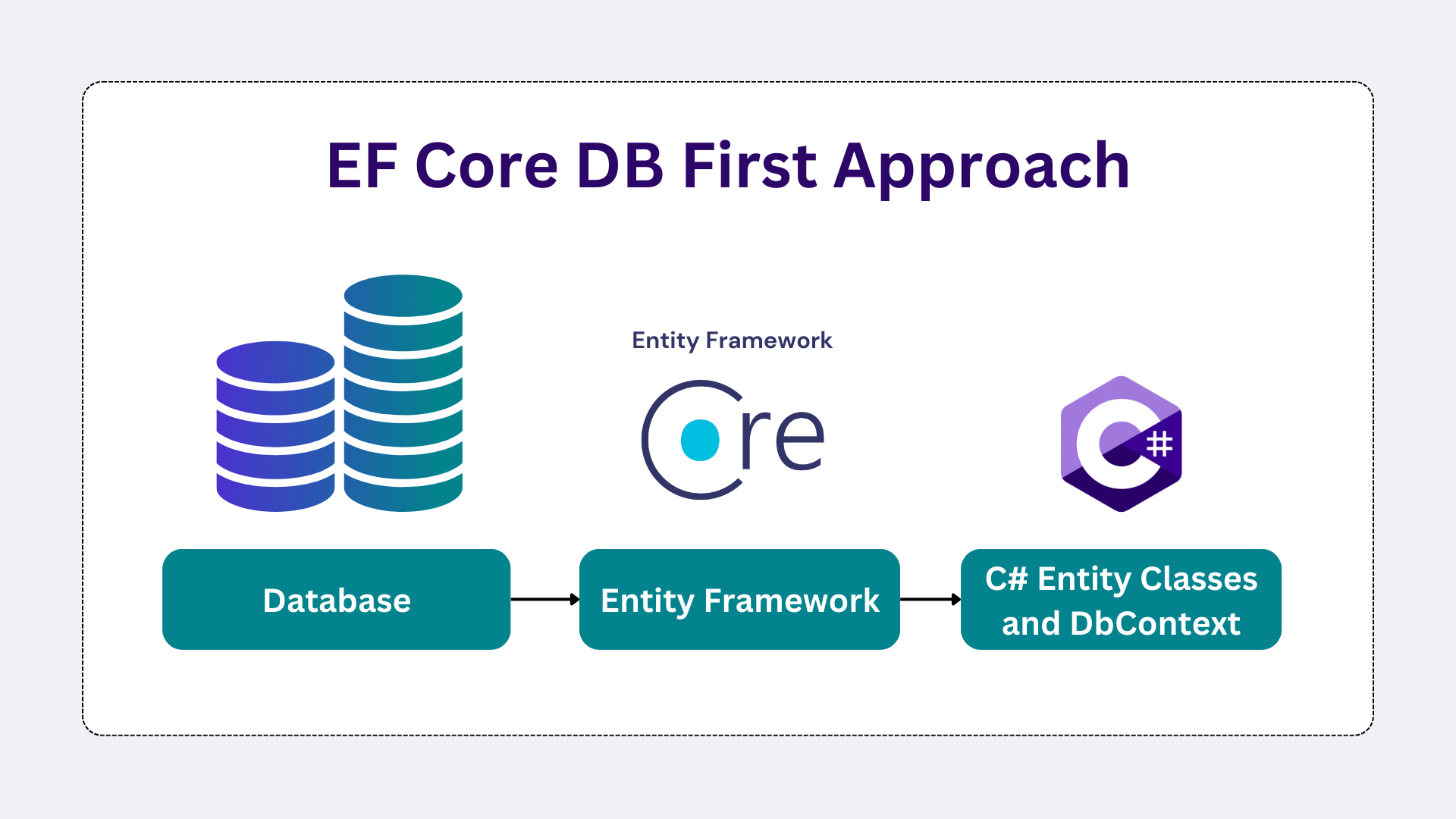